IDE For LLMs
Build, Fine-tune and Deploy like never beforeMastering Model Validation: Effective Train-Test Splits and Cross-Validation Strategies
Learn effective model validation techniques like train-test splits and cross-validation strategies to build robust predictive models. Discover AI best practices and insights tailored for high-stakes industries like banking.
Written by: Greg Michaelson, January 9, 2025
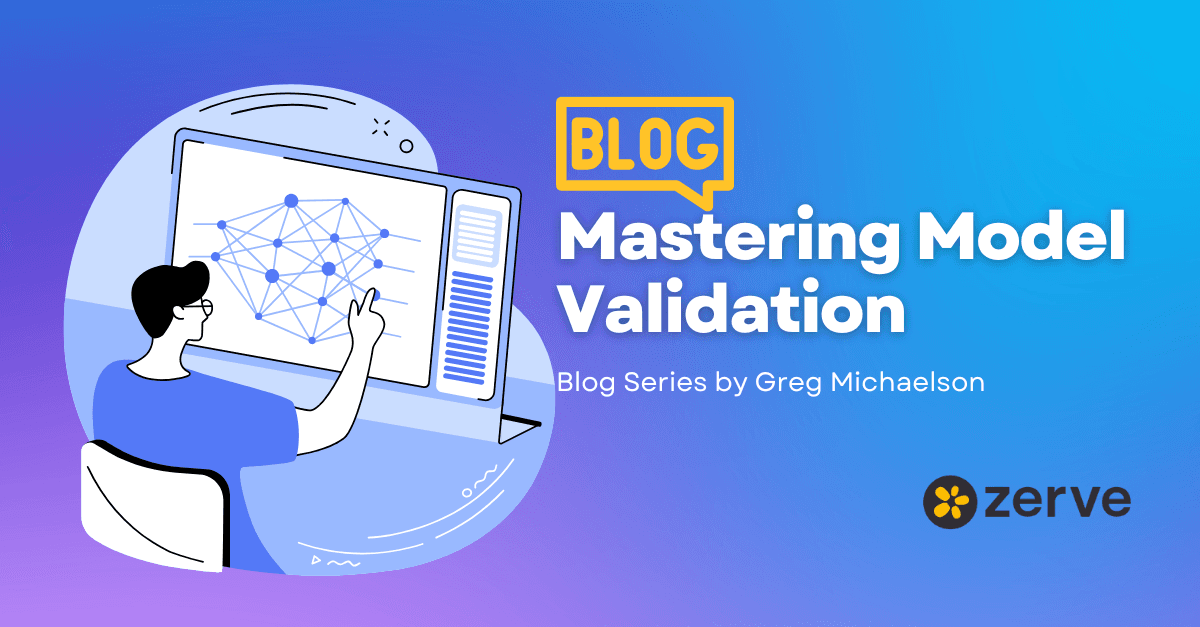
As an experienced data scientist, I’ve learned that one of the most critical steps in building robust predictive models is proper model validation. Whether you're applying supervised learning techniques to classify loan applications or using unsupervised learning to detect anomalies, validation is the cornerstone of ensuring your model generalizes well to unseen data.
In this article, I’ll walk you through the fundamentals of train-test splits and cross-validation strategies, share insights into AI best practices, and discuss their application in the banking sector—a field where accuracy and reliability are paramount.
Why Model Validation Matters
Model validation is more than just a step in the data science best practice workflow; it’s the mechanism that protects us from overfitting and ensures our models deliver meaningful results in real-world applications. Without it, even the most sophisticated model can become a liability.
I remember working with a banking client on a project to predict loan defaults. Initially, the model performed exceptionally well on the training data, with over 95% accuracy. However, when tested on new data, its accuracy plummeted to around 60%. The culprit? Overfitting due to improper validation. This experience taught me the value of robust validation techniques like train-test splits and cross-validation.
The Fundamentals of Train-Test Splits
The train-test split is the simplest and most widely used method of model validation. By dividing the dataset into two parts—a training set and a testing set—we evaluate how well the model generalizes to unseen data.
Here’s how I typically approach a train-test split:
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
# Example dataset: banking transactions for fraud detection
data = pd.DataFrame({
'transaction_amount': [100, 200, 150, 400, 250, 300, 450],
'transaction_time': [5, 12, 8, 20, 15, 25, 30],
'is_fraud': [0, 1, 0, 1, 0, 1, 0]
})
# Features and target
X = data[['transaction_amount', 'transaction_time']]
y = data['is_fraud']
# Train-test split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Training a simple model
model = RandomForestClassifier(random_state=42)
model.fit(X_train, y_train)
# Evaluating the model
accuracy = model.score(X_test, y_test)
print(f"Test Accuracy: {accuracy:.2f}")
This approach provides a quick and effective way to assess model performance. However, its simplicity can also be a limitation, as the results may vary depending on how the data is split. That’s where cross-validation comes in.
Cross-Validation for Robust Model Assessment
Cross-validation divides the data into multiple subsets (folds), training the model on some folds while testing on others. This method gives a more comprehensive evaluation of model performance, reducing variability caused by random splits.
One of my favorite techniques is k-fold cross-validation, particularly when working with critical financial applications like credit scoring models. Here’s how it works:
from sklearn.model_selection import cross_val_score
from sklearn.ensemble import RandomForestClassifier
# Using the same dataset as above
model = RandomForestClassifier(random_state=42)
# Performing k-fold cross-validation
scores = cross_val_score(model, X, y, cv=5) # 5-fold cross-validation
print(f"Cross-Validation Scores: {scores}")
print(f"Mean CV Accuracy: {scores.mean():.2f}")
By using 10-fold cross-validation, we ensured the model was robust across diverse data subsets, giving the client confidence in its reliability.
Another challenge I faced was dealing with imbalanced datasets, which are common in banking. For instance, in fraud detection, the number of fraudulent transactions is often much smaller than the number of legitimate ones. To address this, I’ve used techniques like stratified cross-validation, which ensures each fold in the validation process maintains the same class distribution as the original dataset.
Here’s an example:
from sklearn.model_selection import StratifiedKFold
# Stratified k-fold cross-validation
stratified_kfold = StratifiedKFold(n_splits=5, shuffle=True, random_state=42)
for train_index, test_index in stratified_kfold.split(X, y):
X_train, X_test = X.iloc[train_index], X.iloc[test_index]
y_train, y_test = y.iloc[train_index], y.iloc[test_index]
model.fit(X_train, y_train)
accuracy = model.score(X_test, y_test)
print(f"Fold Accuracy: {accuracy:.2f}")
This approach ensures the model is evaluated on data that reflects real-world conditions, making it more reliable when deployed.
Best Practices for Model Validation
Through my experiences, I’ve identified a few AI best practices for effective model validation:
Always perform multiple validation techniques, such as train-test splits and cross-validation, to ensure consistent results.
Use stratified sampling when working with imbalanced datasets to maintain the integrity of the class distribution.
Regularly evaluate your validation strategy as your dataset evolves or grows.
These practices not only improve model performance but also instill confidence in its predictions, especially in high-stakes industries like banking.
Conclusion
In my work, I’ve seen firsthand how techniques like train-test splits, k-fold cross-validation, and stratified sampling can make the difference between a model that works and one that fails. Validation is not just a step—it’s a practice. By adhering to data science best practices, you’ll build models that not only perform well but also earn the trust of stakeholders.
Try Zerve Now!
Subscribe to our newsletter: